Overview
Hello there, I am Wei Neng. I am currently studying at National University of Singapore (NUS), majoring in Computer Science and specialising in Software Engineering. This portfolio page collates and document my contributions towards the development of the project, LoanBook. It serves to showcase the technical knowledge I have learned throughout the course in CS2103T. My resume and full portfolio can be found here.
LoanBook
Physical management of bicycle loans using pen and paper is hard to organise, unfriendly to the environment and extremely slow. Coupled with the rising competition from bicycle sharing companies such as ofo and MoBike, it is more important than ever for bicycle rental companies to streamline their process and be more efficient to stay competitive.
Introducing LoanBook, the all-in-one free inventory management software that promises to improve the efficiency of your bicycle rental business! It comes out of the box with every feature that your business will require. By parsing commands typed by users, a mouse is no longer required to use the app. Compared to a Graphical User Interface (GUI) or the traditional pen and paper, fast typists will find that LoanBook allows user to manage bicycle loans much faster. LoanBook was built with love together with 4 other students in NUS, namely David Kum, Lim Fong Yuan, Liu Xiaohang and Muhammad Irham.
Noteworthy features includes
-
Managing bicycles statuses
-
Summary report for loan in a given period
-
Email reminding feature to remind users about their pending loan, with future plans for e-receipts
-
Authentication to ensure integrity of the application’s data
Summary of contributions
This section provides a brief summary on the contributions I made for the project. |
-
Major enhancement: Added an authentication feature to improve the integrity of the app’s data.
-
What it does:
-
Allow users to set password and change the password of the app, such that critical comments that affects statistical integrity (Transaction summery & Tax-filing) cannot be performed by unauthorised personnel. Password is also encrypted to prevent hackers from retrieving the raw password.
-
-
Justification:
-
This feature improves the product significantly because now, only authorized users are able use the critical commands (e.g.
delete
command) to delete loans. Without this, the data from the summary report may be tempered with, affecting tax-filling, revenue management.
-
-
Highlights:
-
Allow users to change the password of the app, ensuring that the same password is not used for a long time.
-
Encrypt password using randomly generated salts to provide a higher level of encryption. This ensures that hackers will not be able to reverse engineer the password. Used code by Sergey Kargopolov.
-
Arguments of commands that requires a password do not appear in the command history.
-
Existing commands was modified to require a password input. (E.g.
delete
)
-
-
-
Minor enhancement:
-
Code contributed: RepoSense
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
(4 releases) on GitHub. -
Team Leader of the project, making critical decisions regarding the direction of the project.
-
Added badges to show CI status, code quality and type of licence for the master branch. [#2][#32]
-
Enforced Git discipline across the team, ensuring stability of the master branch.
-
Ensured code quality through reviews on Github.
-
-
Project Enhancement:
-
Documentation:
-
Maintained the issue tracker
-
Changed the gradle build script to display team name: [#1]
-
-
Tools:
-
Integrated TravisCI, AppVeyor, Coveralls, Codacy, and Netlify to the team repo. [#23]
-
Ensures that all code that was pushed adhere to coding standards, and does not break existing code.
-
Automatic deployment of website with Netlify to ensure that team’s web page is always up to date.
-
-
Set up team website.
-
Set up slack notification and web hook for team
-
Provides notification to developers on any updates to the repository.
-
-
-
Contributions to the User Guide
The following sections provides an insight on my ability to write documentations targeting end-users to guide them on the usage of the different features of the app. |
Change the password of the app: setpass
This command changes the current password of the app. This allows you to use a different password in the event that the old password was compromised. Simply follow the example screenshot below:
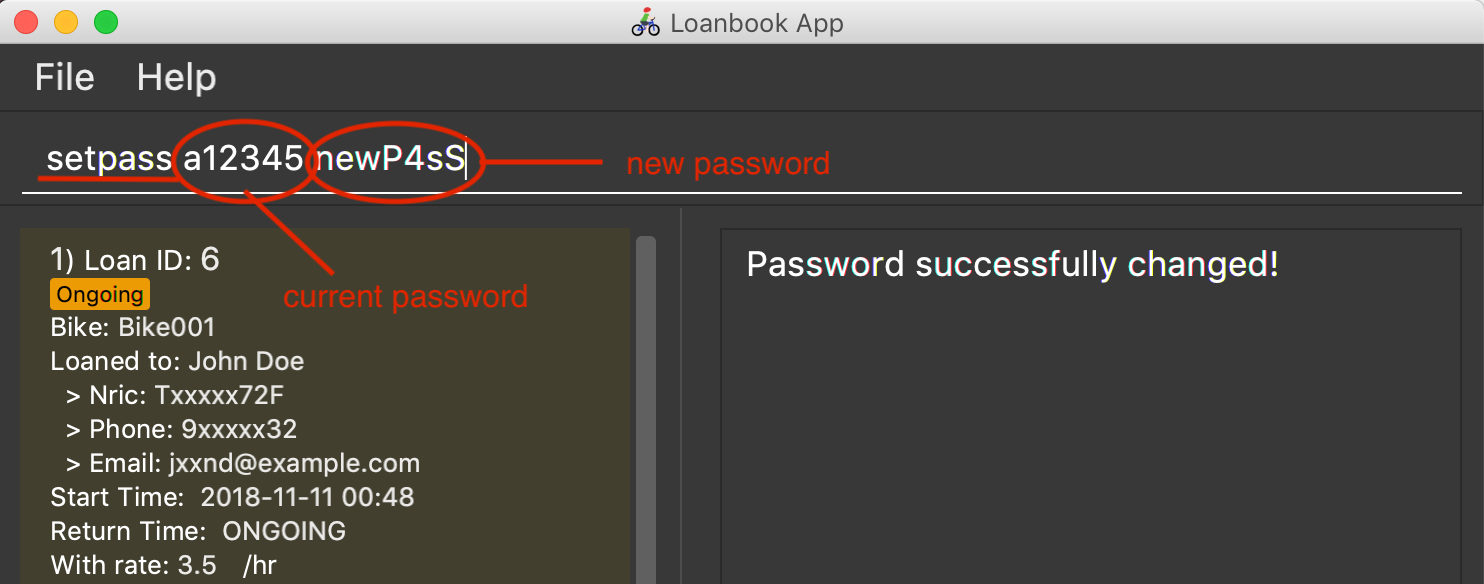
Format: setpass CURRENT_PASSWORD NEW_PASSWORD
List of Parameters:
The old and new passwords of the application.
Note that you only need to use spaces to seperate the two passwords. There is no prefix for this command!
The default password for the app is a12345 . To change the default password, execute the command: setpass a12345 <newpass> .
|
Examples:
-
setpass a12345 n3wP4sS
Set the password of the app ton3wP4sS
.
Locating loans by date of loan: search
Populate all loans that were created between the range provided. If you want to search for loans created within a given period for loan tracking, simply enter the command, as shown in the screenshot below:
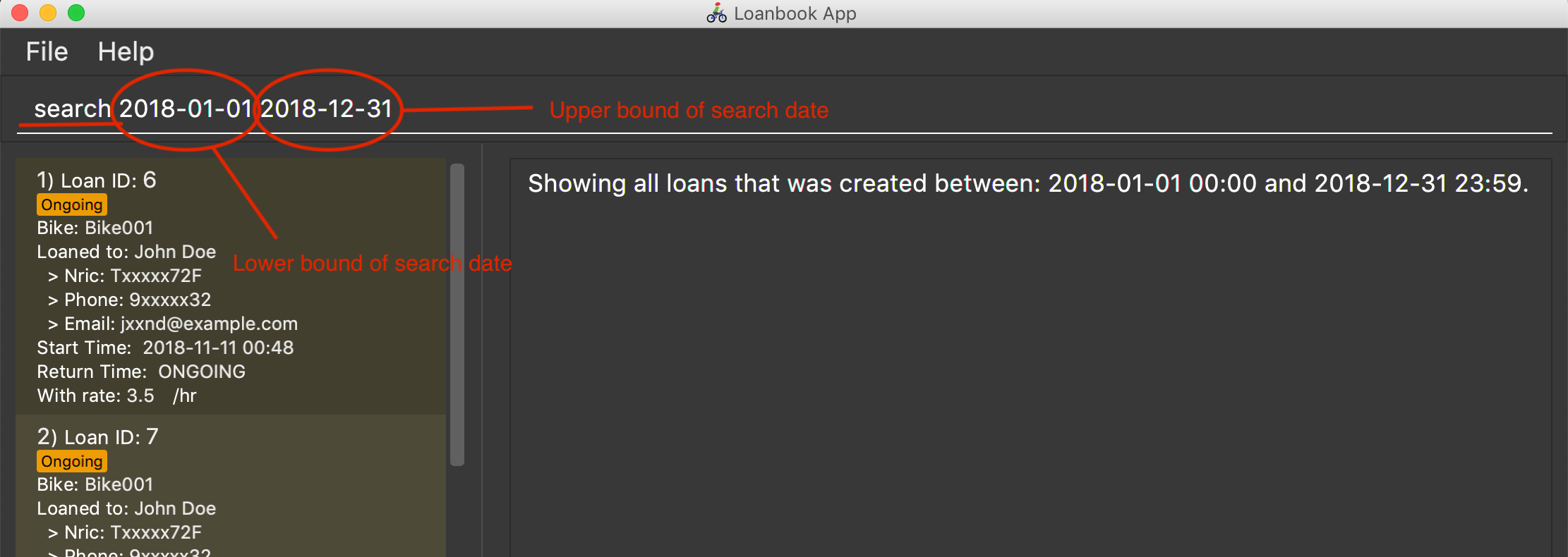
Format: search START_DATE END_DATE
List of Parameters:
START_DATE and END_DATE: The date range in which you want to search for.
Note that you only need to use spaces to seperate the two dates. There is no prefix for this command!
Examples:
-
search 2018-01-01 2018-01-01
Search for loans created on 2018-01-01. -
search 2018-01-01 2018-01-02
Searches for loans created between 2018-01-01 and 2018-01-02, inclusive.
Contributions to the Developer Guide
The following section showcase my ability to write technical documentations to provide future developers with insights on how the application was developed. It also showcase the technical depth of my contributions to the project. |
Authentication
Before critical actions such as deleting a loan can be performed, authentication of user is required. This ensures that only authorized users are able to perform critical actions. This is done by requiring a password before performing a critical action.
Current Implementation
The following provide a walkthrough on how the authentication feature is implemented. |
The authentication mechanism is provided by the Password
class. It implements the following operations that facilitates authentications:
-
Password#isSamePassword(currentPass, providedPass)
— Checks if bothprovidedPass
andcurrentPass
are the same password after decryption. -
Password#generateSalt()
— Generate a hashing salt for the app. -
Password#encrypt(providedPass, salt)
— EncryptprovidedPass
using hashingsalt
.
Given below is an example usage scenario and how the authentication mechanism behaves at each step:
Step 1. The user launches the application for the first time. UserPref
will be initialised with a randomly generated salt by calling Password#generateSalt()
, and a default hashed password from a12345
.
Example of a hashing salt is:
R9hE4pccZYI3BYfQa6etrVD5n7sLRK8G9S8KJRLUqTvdJH15oYnsR1EyrYL2FGH1xDVulTrPEYt1ByVPoVmS4uDDzKzPjZJg2i9SPvOdOMtap6xfBVZLld7ZnEqgdMuhknvLzYilwKy8yHzaEsjk0PEhR5G1XaEMd2dqw6fAhng4ML5UzvIMo2s8HmB4NU7G5pk5yyiQYvam5XrLm0k24ZLUV7zbmmhKXACYlFKcEMMI9LzQcMwBXgN5KHe08H3U
Step 2. The user executes setpass a12345 newP4sS
command to change the password to newP4sS
.
Step 3. Input password will be checked against the app’s password using Password#isSamePassword()
to ensure that the user has sufficient elevation to change the password of the app.
Step 4 Password
class will encrypt the new password using Password#encrypt()
, and call Model#setPass() to changes the password of the application in UserPref
.
Example of an encrypted password:
d46XwZ4pClMXcItYHiNPJ4gHc8IKRFt8QnVDJd8axQ0=
If the current password input is wrong or if the current password is the same as the new password input, it will not call Model#setPass() , so the UserPref state will not be saved.
|
Step 5. Password in UserPref
is saved to the encrypted value of the new password input.
The following sequence diagram shows how the setpass
operation works:
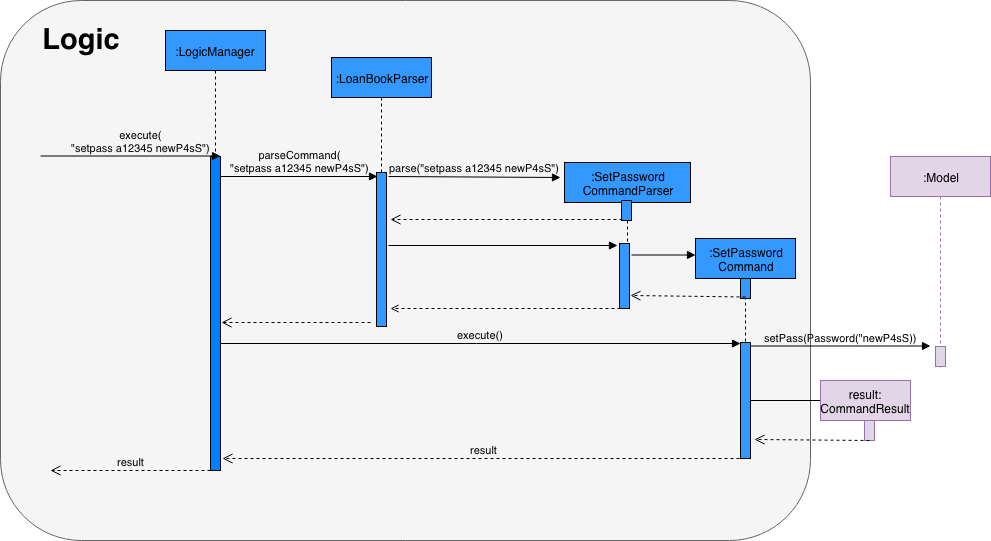
The following activity diagram summarizes what happens when a user executes setpass
:
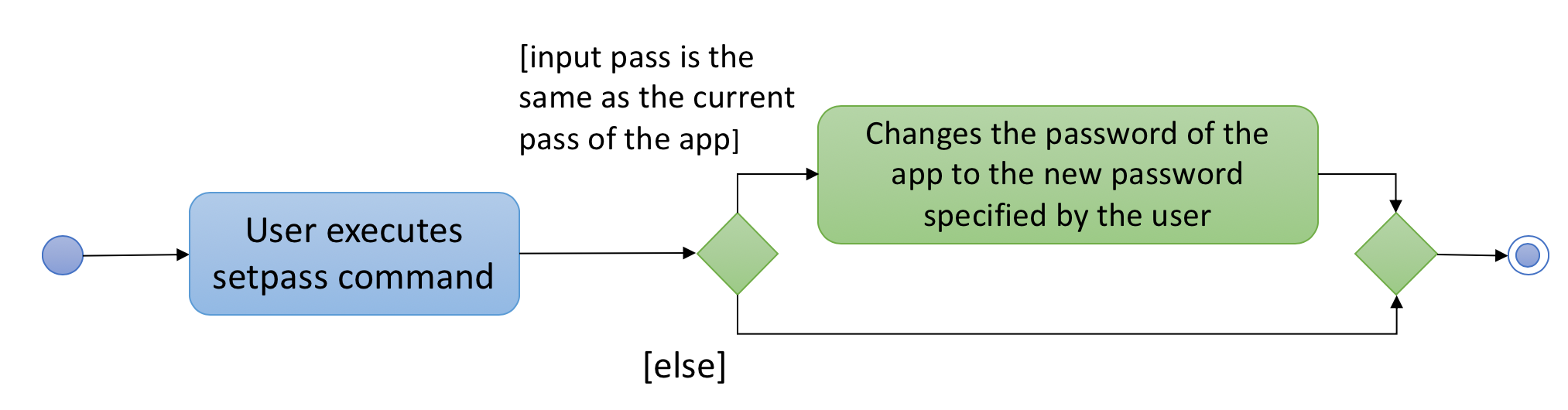
Step 6. The user executes a critical command delete i/1 x/a12345
.
Step 7. This command runs Model#getPass()
to retrieve the current password. It then call Password#isSamePassword()
to determine if the input password in the command is the same as the existing password.
Step 8. Deletion of loan at index 1 will occur.
If current password input is wrong, or if the index provided is invalid, deletion will not occur. |
The following sequence diagram shows how the new delete
operation works:
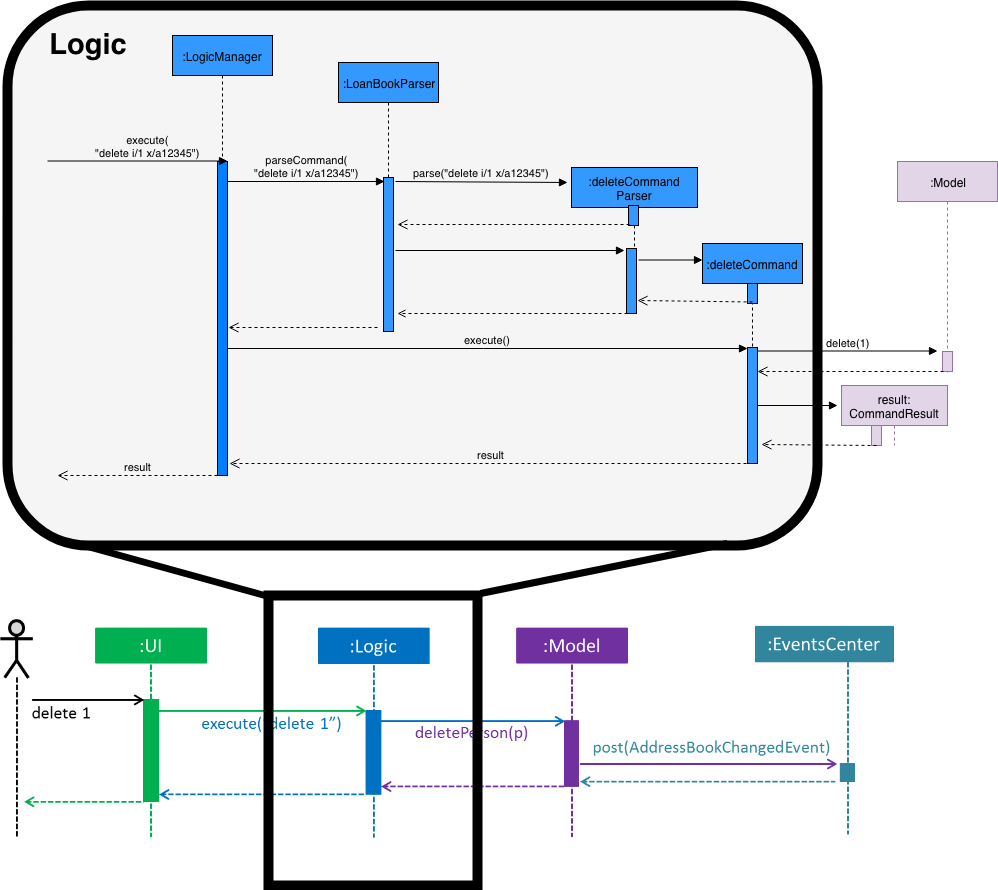
The following activity diagram summarizes what happens when a user executes delete
:
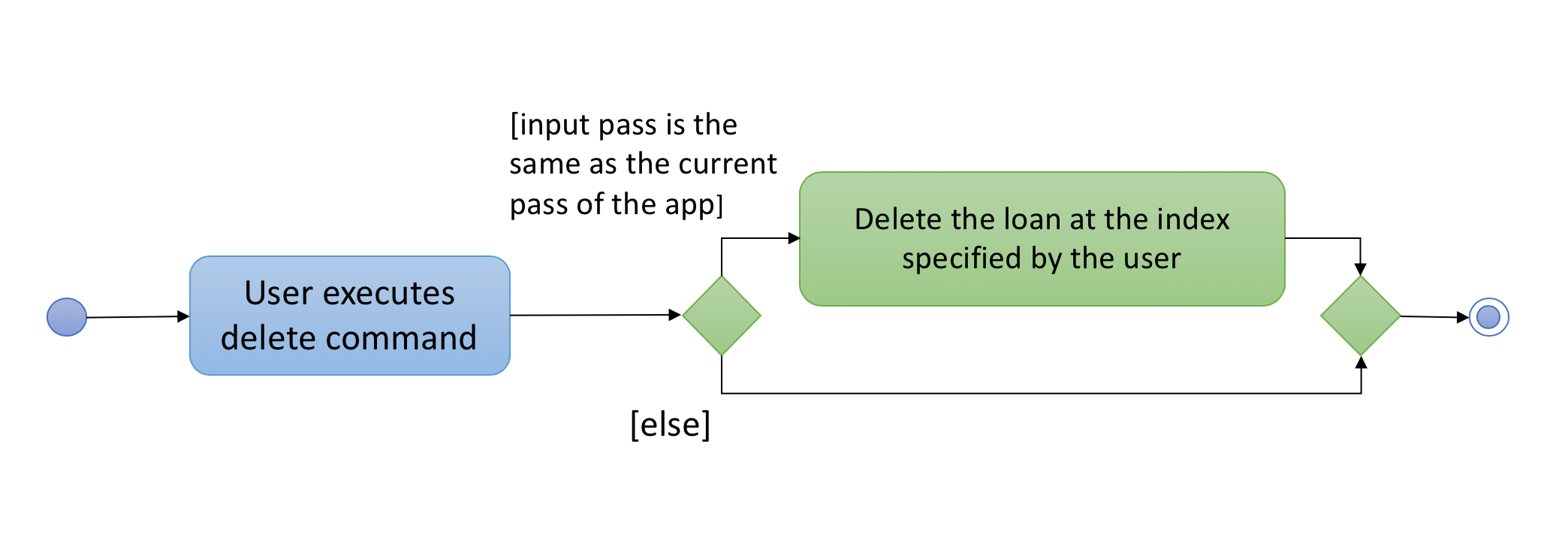
Design Considerations
The following aspects are considerations that our team had made before the implementation of this feature. |
Aspect: How to authenticate users
To ensure that only authorised users can execute elevated commands, we had to consider different alternatives on how to authenticate the user.
-
Alternative 1 (current choice): Require a password every time a critical command is executed
-
Pros:
-
Ensures that each command authenticates the user before executing
-
Easy to implement
-
-
Cons:
-
Might be inconvenient to perform multiple critical commands as repeated typing of password is required.
-
-
-
Alternative 2: A login page to authenticate users
-
Pros:
-
Customized to each staff
-
Provides accountability of each command execution as we can now track which staff ran which commands
-
Scalable for a large company.
-
-
Cons:
-
Difficult to implement
-
Not effective for our target audience as bicycle shop owners are often family-owned business, which does not have a large manpower.
-
If a staff did not log out, non-authorized users will be able to execute critical commands, making the app’s data vulnerable.
-
-
Aspect: Method of encryption
To ensure that the app’s password is properly encrypted, we had to consider between storing the password locally, and storing the password online.
-
Alternative 1 (current choice): Generate a salt to encrypt password, and store the salt locally
-
Pros:
-
Does not require the internet, ensuring that any hack attempts to the app has to be done physically.
-
Much less complicated to implement as compared to alternatives solutions.
-
-
Cons:
-
Encrypted password and salt can be accessed in
preference.json
.
-
-
-
Alternative 2: Send a POST request to an online server to authenticate each login request
-
Pros:
-
If done properly, ensures that hashed password cannot be intercepted/retrieved by hackers.
-
-
Cons:
-
Requires the internet, which might not be available to bicycle shop owners as parks are not fibre-optic ready.
-
Difficult to implement.
-
Data can be intercepted and manipulated during POST request, as opposed to a local storage of password.
-
-